JavaScript SDK in Live Chat
Live Chat offers a JavaScript SDK that enables you to interact with the widget in various ways. Every interaction happens through a globally defined function called liveChat
, which is available anywhere the widget was initialized. The definition of this function in Typescript syntax is as follows:
liveChat(command: string, arg?: any, callback?: (e?: any, r?: any) => any);
Let's take a look at an example. The following snippet will initialize the widget:
liveChat('init', '85ea1938-f457-496d-8532-8fa489beea63', (error, result) => {
if (error) {
console.log('init error: ', error);
} else {
console.log('init result: ', result);
}
});
-
liveChat(...);
is the global function used to interact with the widget. -
'init'
is the first parameter of the function. This is called a command and it tells the widget what you want to do with it. In this case, we instruct the widget to initialize itself. For more commands and details about them, see the Commands (opens in a new tab) section. -
'85ea1938-f457-496d-8532-8fa489beea63'
is the second parameter of the function. This is called a command argument or arg and it provides arguments for the command. A command can have no arguments, an optional argument or a required argument. Both optionality and the type of the argument depend on specific commands. For more details, see the Commands (opens in a new tab) section. -
(error, result) => { ... }˙
is the third parameter of the function. This is a callback function, which will be invoked once the execution of the command finishes. The callback is a function of two parameters - the first parameter represents an error and the second one represents a result. Only one will be defined depending on the execution result. The error parameter will always be defined in case of failure and the result parameter will be defined in case of success.
You can call this function from anywhere in your code where Live Chat has been initialized using the snippet provided on your Infobip account.
Commands
Read the sections below and find out more about the available commands.
Init
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'init' | string | Widget ID | On first invocation | In development |
Example usage
liveChat('init', '85ea1938-f457-496d-8532-8fa489beea63', myInitCallback);
Details
This command should be called as the first one, before any other commands. The argument expects the ID of the widget you would like to initialize. If you would like to initialize with another widget ID without reloading the page, you will have to call either the Clear or the Log out command first.
Auth
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'auth' | string | JWT | YES | In development |
Example usage
liveChat('auth', generateJwt(), myAuthCallback);
Details
This command authenticates the current user using data provided in the JWT. Users authenticated this way are automatically treated as customers in the system. This means that by authenticating, users can verify their identity and access their previous history.
For more info on how to generate the JWT, check this page (opens in a new tab).
Log out
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'logout' | none | N/A | NO | In development |
Example usage
liveChat('logout', null, myLogoutCallback);
Details
This command is used to log out of a currently authenticated user session (this means that the Auth command has to be called first). In addition to logging out of the current session, the browser's local storage entries associated with Live Chat will be cleared and the widget will reload itself. This effectively means that the widget will reset itself to the default state.
Clear
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'clear' | string | Widget ID | OPTIONAL | In development |
Example usage
liveChat('clear', '85ea1938-f457-496d-8532-8fa489beea63', myClearCallback);
Details
This command is very similar to the Log out command. The difference is in the optional Widget ID argument. If the Widget ID is provided, the widget will clear its local storage and attempt to log out the user. If the Widget ID is not provided, the widget will clear its local storage and reload itself to the default state.
Show
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'show' | none | N/A | NO | In development |
Example usage
liveChat('show', null, myShowCallback);
Details
This command simply opens (shows) the widget itself, meaning the widget will expand if it is not expanded yet.
Hide
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'hide' | none | N/A | NO | In development |
Example usage
liveChat('hide', null, myHideCallback);
Details
This command simply hides (closes) the widget itself, meaning the widget will collapse if it is not collapsed yet.
Show launcher
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'show_launcher' | none | N/A | NO | In development |
Example usage
liveChat('show_launcher', null, myShowLauncherCallback);
Details
This command simply shows the widget launcher, but it will not expand the widget itself.
Hide launcher
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'hide_launcher' | none | N/A | NO | In development |
Example usage
liveChat('hide_launcher', null, myHideLauncherCallback);
Details
This command simply hides the widget launcher, which means that if the widget is expanded, it will collapse, and the launcher will also disappear.
Set theme
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'set_theme' | string | Theme name | YES | In development |
Example usage
liveChat('set_theme', 'dark');
Details
This command is useful for applying the theme by theme name. It is important to note that themes must be configured for the widget in the Infobip account, under Channels → Live Chat (opens in a new tab).
Send contextual data
Command | Argument type | Expected argument | Argument required | Callback returns result |
---|---|---|---|---|
'send_contextual_data' | object | Object containing metadata | YES | YES |
Expected argument structure
The argument should be an object with the following fields:
metadata
(REQUIRED)- Object with key-value pairs representing the data you wish to send
- Example:
{ 'seconds_since_last_cart_visit': 178 }
multiThreadStrategy
(OPTIONAL)- String value of either
'ACTIVE'
or'ALL'
'ACTIVE'
will result in metadata being sent only to the currently opened active thread'ALL'
will result in metadata being sent to all threads with currently active conversations
- Used only for multi-threaded widgets
- The default value is
'ACTIVE'
- String value of either
To see what the argument should look like as a whole, see the following Example usage section.
Example usage
liveChat('send_contextual_data', { metadata: {'color': 'green', 'size': 'M' }, multiThreadStrategy: 'ALL' }, myContextualDataCallback);
Details
This command allows you to send contextual data to an existing conversation. In case you call this command before a conversation starts (usually before the user sends the first message), the contextual data request will get queued and executed once the widget receives confirmation of the conversation's creation.
This may cause minor delays between the creation of the conversation and the time it receives the data. If you are expecting to receive contextual data on conversation creation, make sure to take this delay into account.
You can pass any data to a conversation in the key value pairs format. For instance, imagine a clothing store with a Live Chat feature on their website. A customer browsing the site initiates a chat through Live Chat, and all the information about the customer's shopping basket is sent to the agent as the conversation begins. The conversation context card in the agent's interface will automatically show all the shared data, enabling the agent to review it quickly and efficiently.
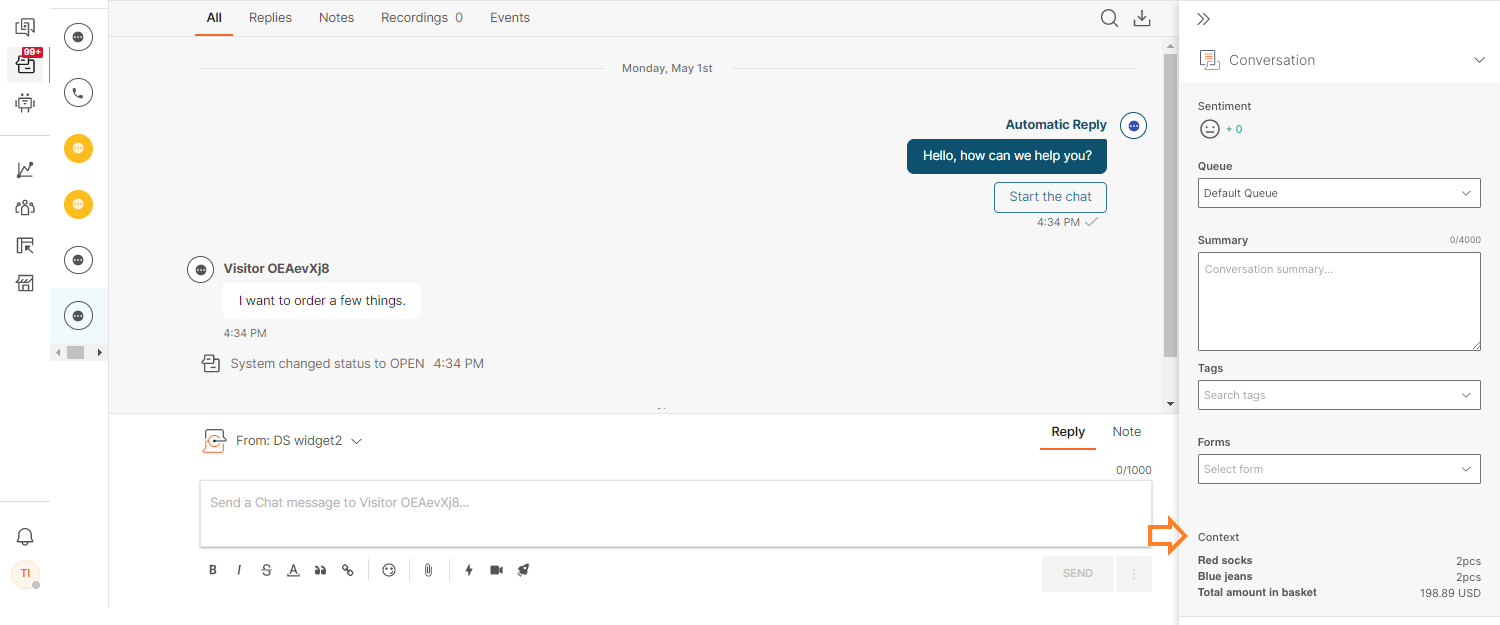