Send a message
After you have defined the message type(s) and channel(s) to use for your communication, use the send a Messages API message (opens in a new tab) to create your request.
Depending on the channels and message type you use, remember to add additional options to your send request.
Validate Messages API request
The Messages API supports request validation using a dedicated endpoint. This endpoint allows you to test your message payload before sending it, ensuring that it meets the necessary requirements for each channel. By validating requests in advance, you can catch potential issues early and receive detailed feedback on resolving them. This feature is particularly useful when dealing with multiple channels in a single request, as each channel has specific requirements. The validation endpoint provides more granular feedback, such as validating messages against standalone API, applying additional checks for each failover step, and flagging unknown fields.
Validation endpoint
This endpoint enables you to perform a detailed validation of Messages API requests. It provides immediate feedback on whether your message payload is valid for submission to the platform. If the request is valid, the endpoint will return 200 OK
. If there are issues, it will return 400 BAD REQUEST
response with information on what needs to be fixed.
To validate your request, follow the steps below:
- Send a
POST
request to the validation endpoint (POST
/resource-management/1/messages/validate
) with your message payload. - If the message passes validation, you will receive a
200 OK
response, indicating it is ready for submission. - If validation fails, you will receive a
400 BAD REQUEST
response with details on the errors.
Sample request
{
"messages": [
{
"channel": "SMS",
"sender": "447491163443",
"destinations": [
{
"to": "111111111"
}
],
"content": {
"body": {
"text": "May the Force be with you.",
"type": "TEXT"
}
}
}
]
}
Example error response
{
"description": "Request cannot be processed.",
"action": "Check the syntax, violations and adjust the request.",
"violations": [
{
"property": "messages[0].content.body",
"violation": "invalid value"
}
]
}
Additional features of endpoint validation:
- Standalone API model validation: Ensure your message conforms to the standards set by each standalone API.
- Failover checks: Verify that each failover step is properly configured.
- Unknown field detection: Flags any fields that could cause issues when sending the request.
The validation endpoint does not send any messages. It only checks the validity of your payload.
For more technical details on endpoint validation, please refer to our API documentation (opens in a new tab).
adaptationMode
The default behavior of the Messages API is now adaptationMode=true
. This means the API will automatically try to adapt messages to fit natively unsupported message types in supported formats of each channel unless explicitly disabled.
With adaptationMode
enabled, the API will adjust the message so it can be sent. However, due to the fact that the API will transform adaptable elements into similar, supported elements, the message can look slightly different.
The adaptationMode
parameter in the Messages API allows you to send and deliver messages even if they contain elements that specified channels do not support.
By default, adaptationMode
is enabled, meaning that the API will automatically adapt messages to fit the capabilities of the recipient channel to ensure successful delivery. The adaptable message elements will get transformed, while non-adaptable elements will be skipped.
Examples of adaptation
- If the header is not supported by the channel, the API will merge the header and body into one message, meaning it will format header as the first line and than new line with the text following.
- If an image is not supported natively, the API will send the URL to it as part of the text instead (for example, it will get added as a plain text in the message).
- If a button is not supported natively by the channel and it cannot be adapted, it will be skipped.
When adaptationMode
is disabled (set as false
), the API does not adapt the message. If the message contains elements that the channel does not support, it will be rejected and will return an error.
The default behavior ensures that messages are delivered whenever possible. However, if you need precise control over message formatting and prefer to receive an error when elements are not supported, you can disable this feature by setting adaptationMode
from true
to false
in your API request.
Example request with adaptationMode
enabled (default behavior)
{
"messages": [
{
"channel": "WHATSAPP",
"sender": "441234567890",
"destinations": [
{
"to": "440987654321"
}
],
"content": {
"body": {
"text": "Thank you for joining us on Athleete Unlocked! Would you like to subscribe for future Athleete events?",
"type": "TEXT"
},
"buttons": [
{
"type": "REPLY",
"text": "Yes",
"postbackData": "true"
},
{
"type": "REPLY",
"text": "No",
"postbackData": "false"
}
]
}
}
]
}
Output
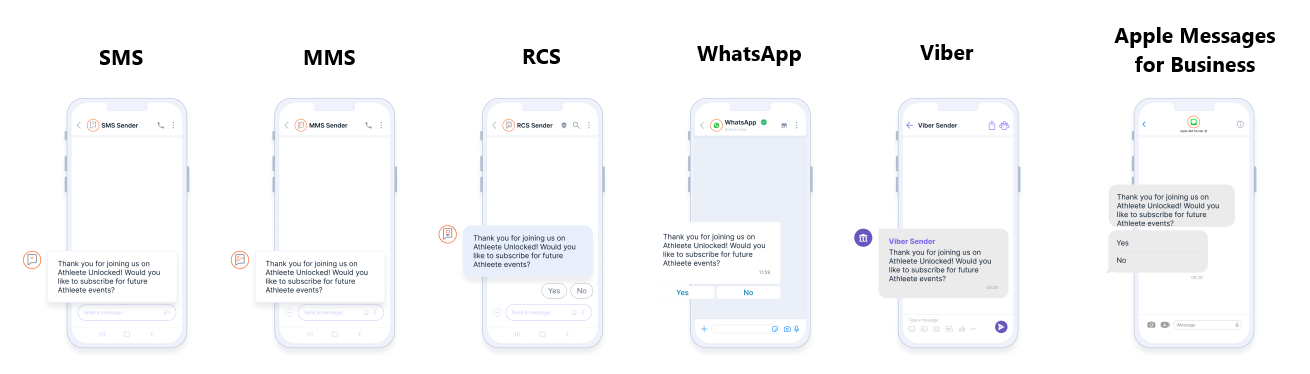
Disabling adaptationMode
If you do not want the API to adapt your messages and would prefer to receive errors when elements are not supported, you need to explicitly set adaptationMode
to false
in your API request.
Example request with adaptationMode
disabled
{
"messages": [
{
"channel": "SMS",
"sender": "441234567890",
"destinations": [
{
"to": "440987654321"
}
],
"content": {
"body": {
"text": "Here is a link to our latest updates!",
"type": "TEXT"
},
"buttons": [
{
"type": "OPEN_URL",
"text": "Visit",
"url": "https://example.com"
}
]
},
"options": {
"adaptationMode": false // Explicitly disabling adaptation
}
}
]
}
In this example, the API will return an error if the channel does not support buttons (for example, SMS).
Supported message types
Messaging channel | Text | Image | Video | Document | Rich link | Auth request | List | Carousel | Templates |
---|---|---|---|---|---|---|---|---|---|
Apple Messages for Business | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ | ✅ | ❌ | ✅* |
Instagram Direct | ✅ | ✅ | 🔄 | 🔄 | ✅ | ❌ | 🔄 | ✅ | ❌ |
LINE | ✅ | 🔄 | 🔄 | 🔄 | ❌ | ❌ | ❌ | ❌ | ❌ |
Messenger | ✅ | ✅ | ✅ | ✅ | ✅ | ❌ | 🔄 | ✅ | ❌ |
MMS | ✅ | ✅ | ✅ | ✅ | 🔄 | ❌ | ❌ | ❌ | ❌ |
RCS | ✅ | ✅ | ✅ | ✅ | ✅ | ❌ | 🔄 | ✅ | ✅* |
SMS | ✅ | 🔄 | 🔄 | 🔄 | ❌ | ❌ | ❌ | ❌ | ❌ |
Viber Bots | ✅ | ✅ | ✅ | ✅ | ✅ | ❌ | 🔄 | ✅ | ❌ |
Viber Business Messages | ✅ | ✅ | ✅ | ✅ | ✅ | ❌ | ❌ | ❌ | ❌ |
✅ | ✅ | ✅ | ✅ | 🔄 | ❌ | ✅ | ❌ | ✅** |
Explanation
âś…: indicates that the channel natively supports the element and the message will be delivered as-is.
❌: indicates that the element is not supported, and an error will be returned (adaptationMode
cannot adapt the element).
🔄: indicates that the channel does not support element natively, but adaptationMode
can convert it (skip/adapt non-supported fields) into a simpler and natively supported format (for example, text or URL).
* for Apple Messages for Business templates and RCS templates, only Text templates are supported.
** for WhatsApp templates, both Text and Carousel templates are supported.
With adaptationMode
set to true
, the API will try to adapt messages to fit the limitations of different messaging channels, ensuring that messages are sent even if the channel does not support all elements. However, in cases of certain strict channel rules and exceptions (for example, button limits and required text), the adaptationMode
will not be able to override them and you will get an error returned.
Buttons
Messaging channel | adaptationMode set to true | adaptationMode set to false |
---|---|---|
Apple Messages for Business | Text Buttons (separate message) | Text Buttons (separate message) |
Instagram Direct | Text with buttons | Text with buttons |
LINE | Text with buttons | Text with buttons |
Messenger | Text with buttons | Text with buttons |
MMS | Text | Error: Buttons not supported |
RCS | Text with buttons | Text with buttons |
SMS | Text | Error: Buttons not supported |
Viber Bots | Text with buttons | Text with buttons |
Viber Business Messages | Text with buttons | Text with buttons |
Text with buttons | Text with buttons |
Image
Messaging channel | adaptationMode set to true | adaptationMode set to false |
---|---|---|
Apple Messages for Business | Image Image caption | Image Image caption |
Instagram Direct | Image Image caption | Image Image caption |
LINE | Image caption URL of the image | Error: Image not supported, but adaptable |
Messenger | Image Image caption | Image Image caption |
MMS | Image Image caption | Image Image caption |
RCS | Image Image caption | Image Image caption |
SMS | Image caption URL of the image | Error: Image not supported, but adaptable |
Viber Bots | Image Image caption | Image Image caption |
Viber Business Messages | Image Image caption | Image Image caption |
Image Image caption | Image Image caption |
Image with header
Messaging channel | adaptationMode set to true | adaptationMode set to false |
---|---|---|
Apple Messages for Business | Message header Image Image caption | Message header Image Image caption |
Instagram Direct | Message header Image Image caption | Message header Image Image caption |
LINE | Message header Image caption URL of the image | Error: Image not supported, but adaptable |
Messenger | Message header Image Image caption | Message header Image Image caption |
MMS | Message header Image Image caption | Message header Image Image caption |
RCS | Message header Image Image caption | Message header Image Image caption |
SMS | Message header Image caption URL of the image | Error: Image not supported, but adaptable |
Viber Bots | Message header Image Image caption | Error: Message header not supported, but adaptable |
Viber Business Messages | Message header Image Image caption | Error: Message header not supported, but adaptable |
Message header Image Image caption | Error: Message header not supported, but adaptable |
Document
Messaging channel | adaptationMode set to true | adaptationMode set to false |
---|---|---|
Apple Messages for Business | Document Document caption | Error: Document filename not supported |
Instagram Direct | Document caption URL of the document | Error: Document not supported, but adaptable |
LINE | Document caption URL of the document | Error: Document not supported, but adaptable |
Messenger | Document | Error: Document caption not supported Error: Document filename not supported |
MMS | Document Document caption | Error: Document filename not supported |
RCS | Document | Error: Document caption not supported Error: Document filename not supported |
SMS | Document caption URL of the document | Error: Document not supported, but adaptable |
Viber Bots | Document (with filename) | Error: Document caption not supported |
Viber Business Messages | Document (with filename) | Error: Document caption not supported |
Document (with filename) Document caption | Document (with filename) Document caption |
Video
Messaging channel | adaptationMode set to true | adaptationMode set to false |
---|---|---|
Apple Messages for Business | Video Video caption | Error: Image preview not supported |
Instagram Direct | Video caption URL of the video | Error: Video not supported, but adaptable |
LINE | Video caption URL of the video | Error: Video not supported, but adaptable |
Messenger | Video | Error: Video caption not supported Error: Image preview not supported |
MMS | Video Video caption | Error: Image preview not supported |
RCS | Video (with image preview) Video caption | Video (with image preview) Video caption |
SMS | Video caption URL of the video | Error: Video not supported, but adaptable |
Viber Bots | Video (with image preview) Video caption | Video (with image preview) Video caption |
Viber Business Messages | Video (with image preview) Video caption | Video (with image preview) Video caption |
Video Video caption | Error: Image preview not supported |
Templates
Messaging channel | adaptationMode set to true | adaptationMode set to false |
---|---|---|
Apple Messages for Business | Template | Template |
Instagram Direct | Not supported | Not supported |
LINE | Not supported | Not supported |
Messenger | Not supported | Not supported |
MMS | Not supported | Not supported |
RCS | Template | Template |
SMS | Not supported | Not supported |
Viber Bots | Not supported | Not supported |
Viber Business Messages | Not supported | Not supported |
Template | Template |
Failover
User failover to always deliver your messages. This option provides messages delivery on other channels in case first option was unsuccessful.
You can:
- Automate channel switching: Messages API automatically changes channel in the event of a failed messages delivery.
- Configure validity period: Define period from 10 seconds to 48 hours for each channel. After validity period is expired, channel is switched and validity period for new channel starts.
- Customize failover flow: Define and prioritize channel failover order.
Message status reports
Use the webhooks option to set the URL where you want to receive message status reports. Define the URL under the webhooks.delivery.url
parameter for delivery reports or webhooks.seen.url parameter
for seen reports in the API request. Note that seen reports are not supported by all channels.
{
"messages": [
{
"channel": "WHATSAPP",
"sender": "441234567890",
"destinations": [
{
"to": "440987654321"
}
],
"content": {
"body": {
"text": "Thank you for your registration!",
"type": "TEXT"
}
},
"webhooks": {
"delivery": {
"url": "https://notifyme.delivery"
},
"seen": {
"url": "https://notifyme.seen"
}
}
}
]
}
Tracking messages with callbackData
The callbackData
parameter can store additional data used for identifying, managing, or monitoring a message. It increases the versatility of Messages API by allowing you to attach and track custom data to messages that then reflects in the Seen and Delivery reports.
The parameter can be associated with both outbound and an incoming messages:
- Outbound (MT) message: If
callbackData
is specified when sending a message, this value will be attached to the message. - Incoming (MO) message: If
callbackData
is defined in the setup for handling incoming messages, this value will be used.
Campaign reference ID
The campaign reference ID is a user-defined identifier that you can assign to each communication campaign within each API call at the message level. It allows you to gain enhanced campaign management capabilities, improved data insights, and streamlined processes for optimizing campaign performance:
- Tracking and management: Efficiently track and manage the performance of your communication campaigns. You can gain detailed insights into various success metrics by assigning a unique ID to each campaign.
- Aggregated overview: Compile and view aggregated data on your performance across different channels.
- Metrics API integration: Campaign Reference ID is integrated into our Metrics API. This integration provides you access to detailed delivery reports and simplifies the analysis of campaign performance metrics.
URL tracking and shortening
This feature enables you to shorten the URLs and monitor click activity to assess engagement and message effectiveness:
- Shorten URLs: Use the
shortenUrl
option to create short, clean URLs. - Track clicks: Utilize the
trackClicks
option to monitor click activity on sent URLs. - Custom tracking URL: Specify a custom
trackingUrl
for enhanced tracking. - Protocol removal: Remove the protocol from URLs with the
removeProtocol
option. - Custom domain: Use the
customDomain
option for branded shortened URLs.
To find out more on how to use the URL tracking and shortening feature, go to our API documentation on sending a Messages API message (opens in a new tab).
Scheduling
The scheduling feature in Messages API allows you to schedule and automate the sending of messages within a channel. This feature allows you to specify the content, timing and target channels, providing a convenient and effective way to manage communications. You can, for example, schedule a promotional message to be sent at optimal times for maximum engagement.
Scheduling scenarios:
- Single channel message: Scheduling triggers the message sending.
- Failover: Scheduling initiates the message from the first listed channel, with subsequent failover scenarios following the set failover and validity logic.