Reach the World
Enrich your application with easy-to-integrate, secure communication APIs offering channels your customers widely use and love.
import { Infobip, AuthType } from '@infobip-api/sdk';
const infobipClient = new Infobip({
baseUrl: process.env.INFOBIP_URL,
apiKey: process.env.INFOBIP_KEY,
authType: AuthType.ApiKey,
});
try {
const infobipResponse = await infobipClient.channels.sms.send({
type: 'text',
messages: [{
destinations: [
{
to: '447123456789',
},
],
from: 'Infosum SDK Test',
text: 'Hello World',
}],
});
} catch (error) {
console.error(error);
}
from infobip_channels.sms.channel import SMSChannel
def main():
channel = SMSChannel.from_auth_params(
{"base_url": "<your_base_url>", "api_key": "<your_api_key>"}
)
sms_response = channel.send_sms_message(
{
"messages": [
{
"destinations": [{"to": "<phone_number>"}],
"from": "InfobipSMS",
"text": "Hi! I'm your first Infobip message. Have a lovely day!",
}
]
}
)
print(sms_response)
if __name__ == "__main__":
main()
import com.infobip.ApiClient;
import com.infobip.ApiException;
import com.infobip.ApiKey;
import com.infobip.BaseUrl;
import com.infobip.api.SmsApi;
import com.infobip.model.SmsAdvancedTextualRequest;
import com.infobip.model.SmsDestination;
import com.infobip.model.SmsTextualMessage;
import java.util.List;
/**
* Requires JDK 11 or above. Dependency declaration (Maven example):
* <dependency>
* <groupId>com.infobip</groupId>
* <artifactId>infobip-api-java-client</artifactId>
* <version>4.0.0</version>
* </dependency>
*/
public class Infobip {
public static void main(String[] args) {
var client = ApiClient.forApiKey(ApiKey.from("<your API key>"))
.withBaseUrl(BaseUrl.from("<your base URL"))
.build();
var api = new SmsApi(client);
var message = new SmsTextualMessage()
.from("InfoSMS")
.destinations(List.of(new SmsDestination().to("<your destination>")))
.text("Hello World from infobip-api-java-client!");
var request = new SmsAdvancedTextualRequest()
.messages(List.of(message));
try {
var response = api.sendSmsMessage(request).execute();
System.out.println(response);
} catch (ApiException exception) {
System.out.printf("Received status %d when calling the Infobip API.%n", exception.responseStatusCode());
if (exception.details() != null) {
System.out.printf("Error details: %s%n", exception.details().getText());
}
}
}
}
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://%7BbaseUrl%7D/sms/2/text/advanced" method := "POST" payload := strings.NewReader(`{"messages":[{"destinations":[{"to":"41793026727"}],"from":"InfoSMS","text":"This is a sample message"}]}`) client := &http.Client { } req, err := http.NewRequest(method, url, payload) if err != nil { fmt.Println(err) return } req.Header.Add("Authorization", "{authorization}") req.Header.Add("Content-Type", "application/json") req.Header.Add("Accept", "application/json") res, err := client.Do(req) if err != nil { fmt.Println(err) return } defer res.Body.Close() body, err := ioutil.ReadAll(res.Body) if err != nil { fmt.Println(err) return } fmt.Println(string(body))
}
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://%7BbaseUrl%7D/sms/2/text/advanced',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS =>'{"messages":[{"destinations":[{"to":"41793026727"}],"from":"InfoSMS","text":"This is a sample message"}]}',
CURLOPT_HTTPHEADER => array(
'Authorization: {authorization}',
'Content-Type: application/json',
'Accept: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
var client = new RestClient("https://{baseUrl}/sms/2/text/advanced");
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AddHeader("Authorization", "{authorization}");
request.AddHeader("Content-Type", "application/json");
request.AddHeader("Accept", "application/json");
var body = @"{""messages"":[{""destinations"":[{""to"":""41793026727""}],""from"":""InfoSMS"",""text"":""This is a sample message""}]}";
request.AddParameter("application/json", body, ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
require "uri"
require "json"
require "net/http"
url = URI("https://{baseUrl}/sms/2/text/advanced")
https = Net::HTTP.new(url.host, url.port)
https.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Authorization"] = "{authorization}"
request["Content-Type"] = "application/json"
request["Accept"] = "application/json"
request.body = JSON.dump({
"messages": [
{
"destinations": [
{
"to": "41793026727"
}
],
"from": "InfoSMS",
"text": "This is a sample message"
}
]
})
response = https.request(request)
puts response.read_body
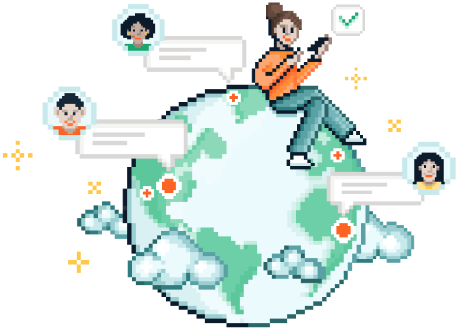
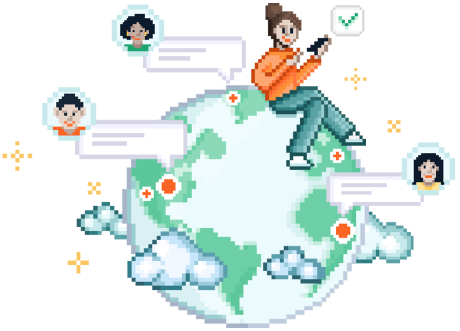
SMS
Simply send anywhere
Send and receive SMS messages with just a few lines of code. View logs and track delivery receipts while we handle the complexity of mobile operators, network logic, and local regulations.
Try it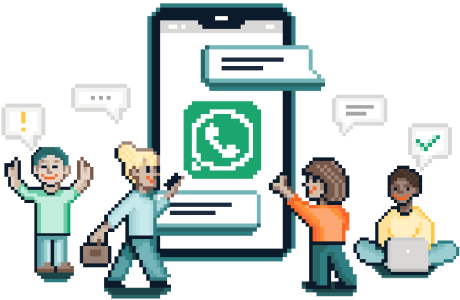
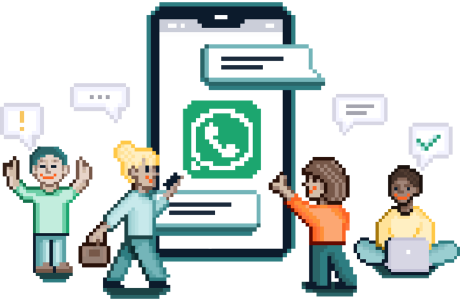
Engage with rich content
Use our WhatsApp Business API to send and receive simple text or engage your audience with interactive buttons, lists, and rich media. Automate your messaging with easy-to-use templates.
Try it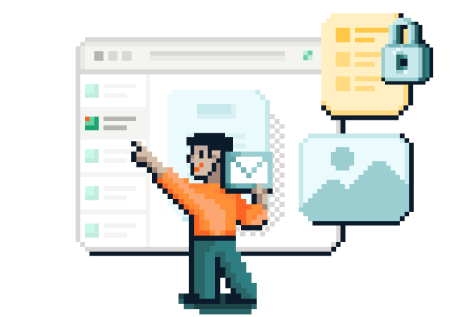
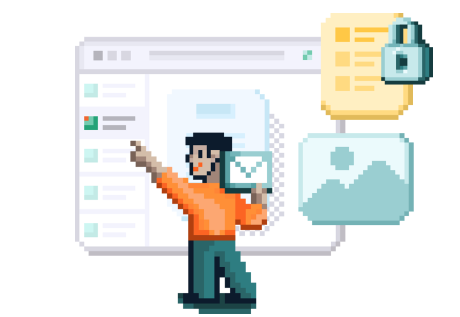
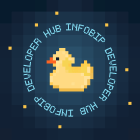
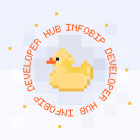

Tools and SDKs





Shopify for people
Integrate Shopify data with Infobip to communicate with customers across multiple channels.
Read more
Slack messaging
Integrating Slack with Infobip Conversations platform lets you move messages into Slack channels.
Read more
Zapier messaging
Connect Zapier with the Infobip platform for the SMS, Voice, and Viber communication channels.
Read more